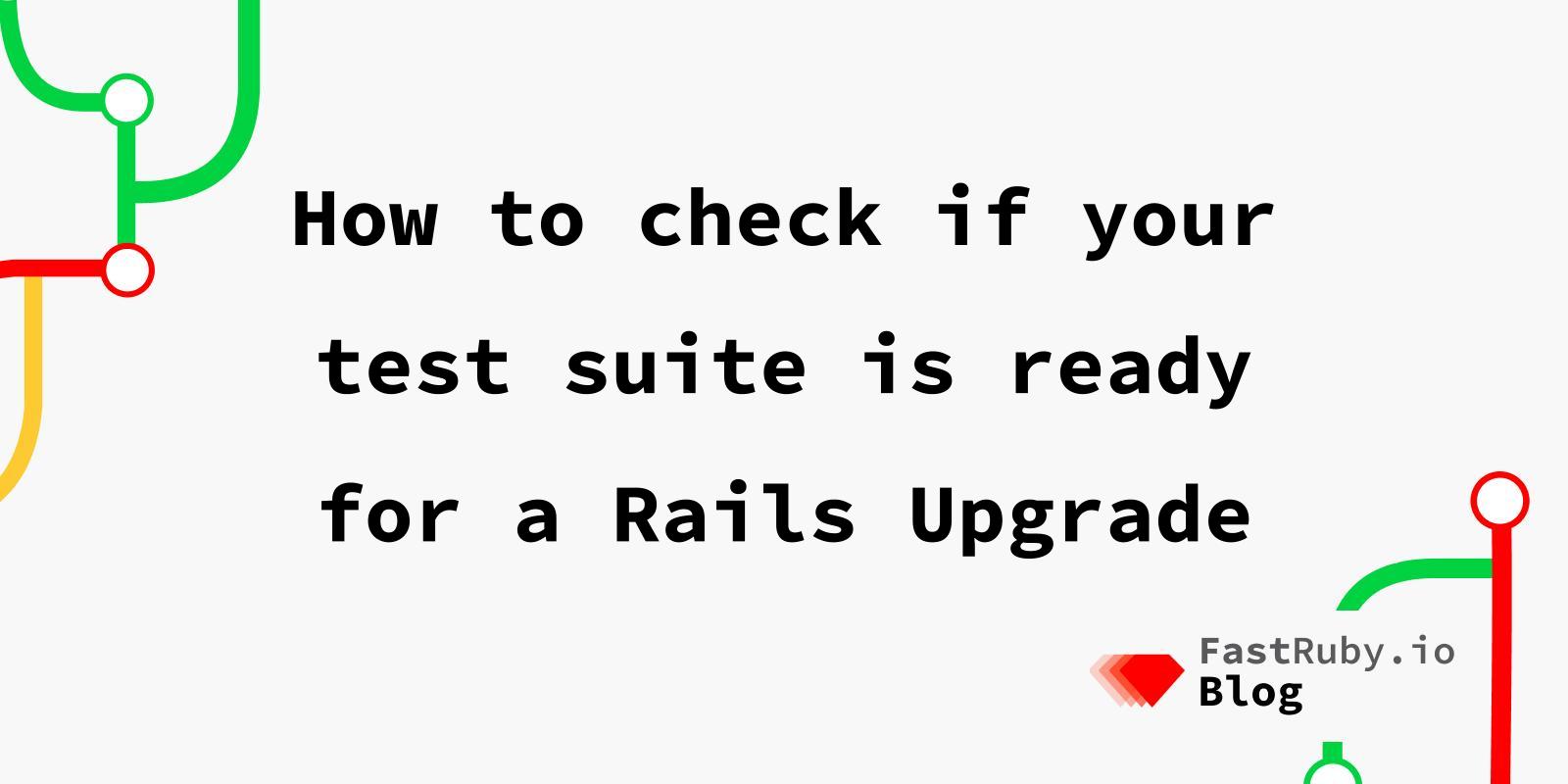
How to check if your test suite is ready for a Rails Upgrade
Having a clear idea of how much test coverage your Rails application has is really important. Especially if you are planning to upgrade to a newer version of Rails. A good test suite will tell you if your application is working as it did before the upgrade.
At FastRuby.io , we recommend having at least 80% of your application covered before attempting to upgrade. A number lower than that would require you to make a lot more manual testing to ensure that the application is properly working after the upgrade. If your application doesn’t meet that number, we suggest to first spend some time improving the test suite before starting the upgrade.
In this article, I’ll show you how you can measure your test coverage using SimpleCov .
A quick note before starting: SimpleCov doesn’t work in projects with Ruby 1.8.7
or lower. If that’s your case, you can try rcov .
Setup
- Add the gem to your Gemfile:
# Gemfile
gem 'simplecov', require: false, group: :test
- Add the configuration to your test helper:
# test/test_helper.rb (minitest) / spec_helper.rb (rspec)
if ENV['COVERAGE'] == 'true'
require 'simplecov'
SimpleCov.start 'rails'
end
In order to see the report that SimpleCov generates, you’ll have to run your entire test suite. You can opt for generating the report locally or in your CI service.
Running SimpleCov locally
This is the most common way to use SimpleCov. It works perfect if you already have the application set up on your computer.
- Install the gem:
$ bundle install
- Run your test suite with the
COVERAGE=true
flag:
(e.g.) $ COVERAGE=true rspec spec
- Open the report generated:
$ open coverage/index.html
It should look something like this:
Running SimpleCov in the CI
There are times when it’s easier to run SimpleCov in your CI service instead of locally. Maybe because you don’t have the application set up on your computer or because the test suite takes too long to run.
Most continuous integration services (e.g. CircleCI ) have the option to enable parallelism. This option breaks up your test suite into several parts and runs it in different containers for a faster execution. The issue with this is that SimpleCov will generate a separate result for each of your containers, which is not ideal. Luckily there is an easy solution for that.
SimpleCov.collate
allows you to fetch all the containers and merge them into a single result set. This can be added to a rake task for example:
# lib/tasks/coverage_report.rake
namespace :coverage do
desc "Collates all result sets generated by the different test runners"
task :report do
require 'simplecov'
SimpleCov.collate Dir["simplecov-resultset-*/.resultset.json"]
end
end
To generate the report you will need to install the gem and run the tests inside the configuration file of your CI service (e.g. .circleci/config.yml
). If you have parallelism enabled, you will also need to run the rake task that merges the results: rake coverage:report
.
Once the CI finishes running, the report should be visible in the files section (e.g. artifacts ) of your CI service.
The SimpleCov.collate
method is fairly recent . In the past we had to do it manually. We wrote an article about it if you want to check it out.
Conclusion
SimpleCov is a really handy tool for Rails Upgrades. We use it every time we work on a Roadmap project. I hope you use it too after reading this article.
Finally, if you need help upgrading your Rails application, check out our free eBook: The Complete Guide to Upgrade Rails .