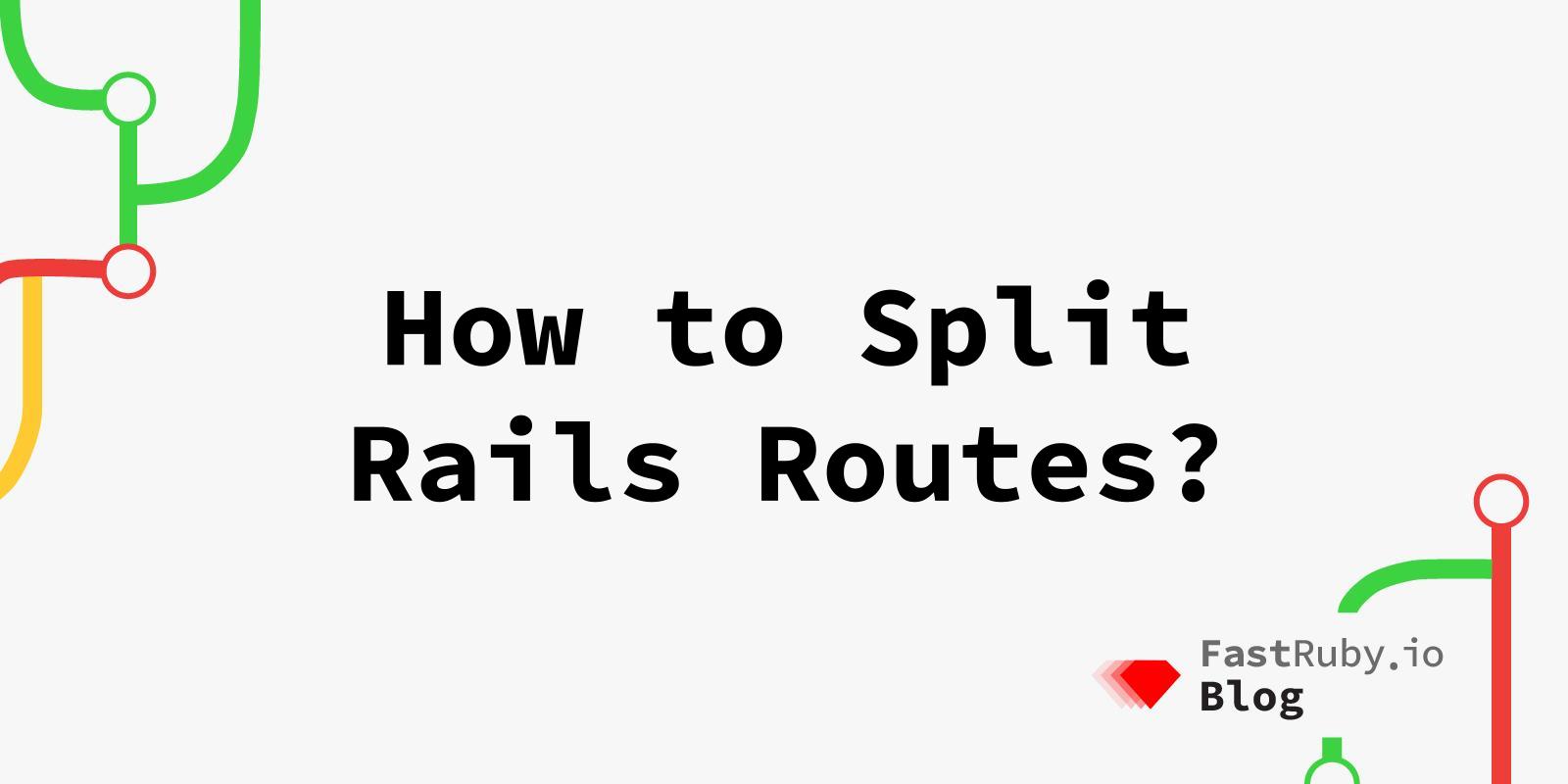
How to Split Rails Routes?
In this article I’ll discuss a strategy for different readability: a simple way to split your routes file on a Ruby on Rails project.
Usually, when we work on large Ruby on Rails applications, those come with a huge routes file in the config/routes.rb
.
This can lead to a bad developer experience or a “your file has too many lines” linter warning.
Before jumping into code examples, it’s important to note that the processing order in routes.rb
is top to bottom.
Rails 6.1 brought back the feature that allows you to load external routes file from the router, as it was removed in 2012.
Check out your project’s version to make sure it’s compatible with this simple solution below.
Let’s say we have our project with this structure:
Controllers folder example
Ideally this project will have namespaced controllers structured in routes like this:
# config/routes.rb
Rails.application.routes.draw do
root to: "home#index"
devise_for :users
namespace :api do
# resources
end
namespace :admin do
# resources
end
namespace :blog do
# resources
end
end
Keeping a nice and useful rails-way of interpretation, these routes file can be easily split using high-level namespaces into other files.
# config/routes/admin.rb
namespace :admin do
# resources
end
# config/routes/api.rb
namespace :api do
# resources
end
# config/routes/blog.rb
namespace :blog do
# resources
end
And finally:
# config/routes.rb
Rails.application.routes.draw do
root to: "home#index"
devise_for :users
draw(:admin) # Will load route file located in `config/routes/admin.rb`
draw(:api) # Will load route file located in `config/routes/api.rb`
draw(:blog) # Will load route file located in `config/routes/blog.rb`
end
Older Rails versions
If you’re running your rails project at 2.x version your router probably uses ActionController::Routing
to draw your routes. At rails 3.0 or higher the router drawer will be defined by each application namespaced within your Application module.
if Rails::VERSION::MAJOR > 2
YourAppName::Application.routes.draw do
...
end
else
ActionController::Routing::Routes.draw do |map|
...
end
end
You can use this quote if you’re on a dual boot .
It’s possible your project might need this to load external files:
# application.rb
config.paths["config/routes"] += Dir[Rails.root.join("config/routes/*.rb")]
Rails docs does not recommend route splitting, unless you really need it. It’s up to your project to decide how long files should be and how routes file should be listed. Developers will have different experiences and different points of view about this, check with your team to see what they think about this.